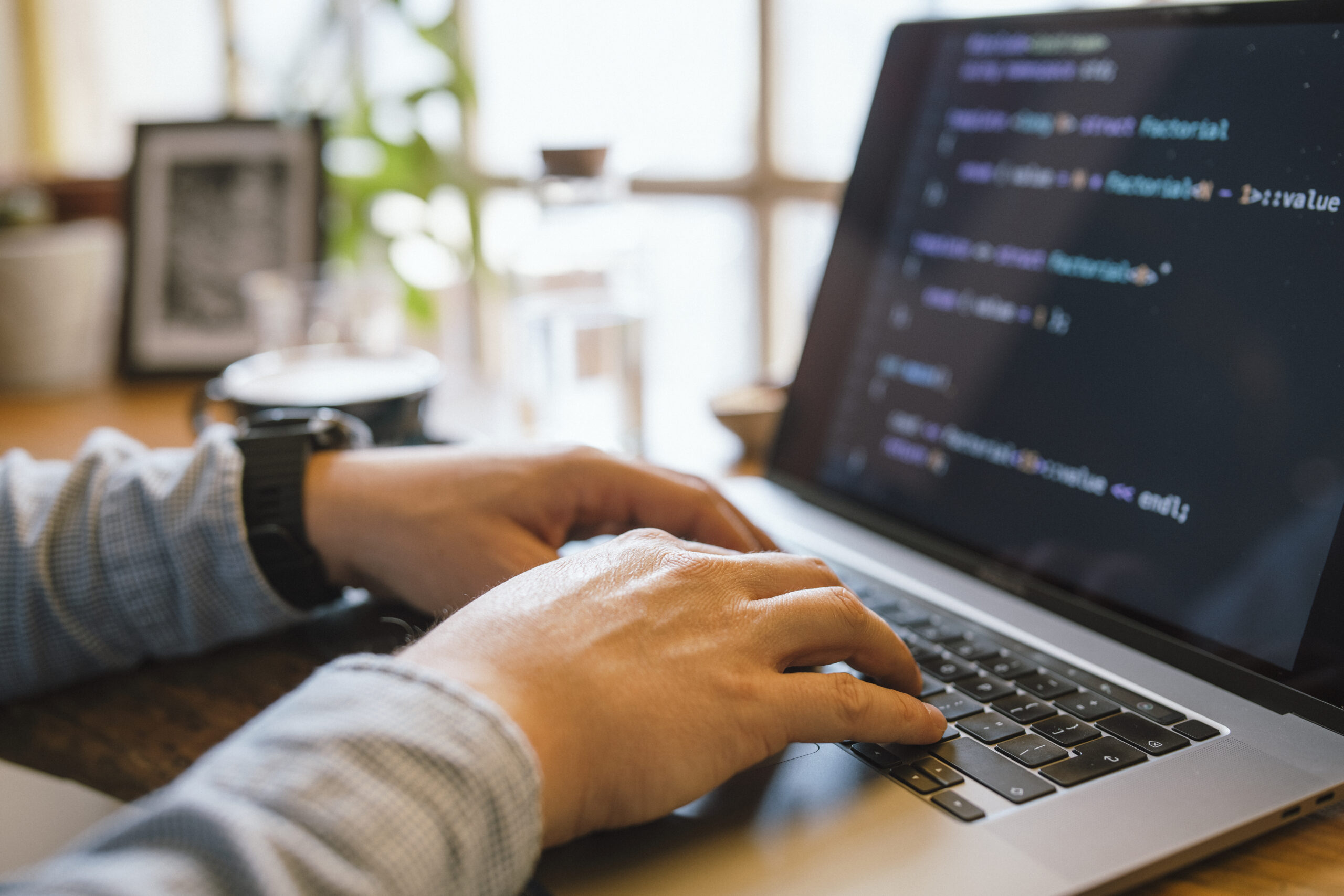
Debugging is one of the most important — still usually neglected — competencies in a developer’s toolkit. It isn't nearly repairing damaged code; it’s about being familiar with how and why things go Incorrect, and Understanding to Feel methodically to resolve complications competently. Whether you're a beginner or a seasoned developer, sharpening your debugging skills can preserve hrs of disappointment and drastically boost your productivity. Listed here are many techniques to aid developers amount up their debugging activity by me, Gustavo Woltmann.
Learn Your Applications
Among the list of fastest techniques developers can elevate their debugging competencies is by mastering the instruments they use every day. Though producing code is one particular Portion of development, recognizing tips on how to connect with it properly in the course of execution is Similarly significant. Modern day improvement environments come Geared up with effective debugging capabilities — but quite a few developers only scratch the area of what these applications can do.
Take, one example is, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, stage by code line by line, and also modify code within the fly. When made use of accurately, they let you observe accurately how your code behaves for the duration of execution, which is a must have for tracking down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for front-finish builders. They enable you to inspect the DOM, monitor network requests, watch genuine-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, resources, and network tabs can switch disheartening UI concerns into workable responsibilities.
For backend or program-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Handle about running processes and memory administration. Studying these equipment could possibly have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into snug with version Handle programs like Git to be familiar with code history, uncover the precise minute bugs were being released, and isolate problematic changes.
In the end, mastering your resources usually means likely further than default configurations and shortcuts — it’s about creating an intimate knowledge of your improvement natural environment to make sure that when challenges crop up, you’re not shed at the hours of darkness. The greater you realize your resources, the more time you are able to invest solving the particular trouble instead of fumbling via the process.
Reproduce the Problem
One of the most significant — and infrequently forgotten — ways in helpful debugging is reproducing the situation. In advance of jumping in to the code or making guesses, builders need to produce a regular surroundings or scenario where by the bug reliably seems. Without having reproducibility, repairing a bug gets to be a game of probability, typically leading to squandered time and fragile code alterations.
Step one in reproducing an issue is accumulating just as much context as you possibly can. Talk to inquiries like: What actions led to The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you may have, the much easier it turns into to isolate the precise problems under which the bug happens.
Once you’ve gathered adequate information, try and recreate the issue in your neighborhood environment. This might mean inputting a similar info, simulating identical consumer interactions, or mimicking process states. If the issue seems intermittently, take into consideration producing automated exams that replicate the sting cases or condition transitions associated. These tests not merely help expose the challenge but will also avoid regressions Sooner or later.
Sometimes, the issue can be surroundings-precise — it'd occur only on specified functioning systems, browsers, or below individual configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the challenge isn’t just a stage — it’s a attitude. It calls for endurance, observation, in addition to a methodical approach. But when you can constantly recreate the bug, you might be now midway to correcting it. With a reproducible scenario, You should use your debugging resources additional proficiently, exam opportunity fixes properly, and connect extra Evidently with all your group or customers. It turns an abstract criticism right into a concrete problem — and that’s in which developers prosper.
Read through and Recognize the Error Messages
Error messages tend to be the most valuable clues a developer has when something goes wrong. Instead of seeing them as frustrating interruptions, builders really should understand to deal with error messages as direct communications from the procedure. They generally let you know just what happened, where by it took place, and from time to time even why it took place — if you know how to interpret them.
Start by looking at the concept carefully As well as in total. Several developers, specially when below time tension, look at the very first line and straight away start off generating assumptions. But deeper from the error stack or logs may perhaps lie the real root trigger. Don’t just duplicate and paste error messages into search engines like google and yahoo — browse and recognize them first.
Split the error down into areas. Is it a syntax error, a runtime exception, or a logic mistake? Does it position to a specific file and line variety? What module or function activated it? These questions can information your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally stick to predictable styles, and Studying to recognize these can greatly quicken your debugging approach.
Some faults are vague or generic, and in These situations, it’s very important to examine the context during which the mistake happened. Check connected log entries, input values, and recent alterations from the codebase.
Don’t overlook compiler or linter warnings either. These usually precede much larger challenges and provide hints about probable bugs.
Finally, error messages aren't your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, encouraging you pinpoint issues quicker, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When used effectively, it provides real-time insights into how an software behaves, helping you understand what’s happening underneath the hood without having to pause execution or step through the code line by line.
A good logging strategy starts with knowing what to log and at what amount. Popular logging degrees include things like DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for comprehensive diagnostic info throughout development, Facts for normal situations (like thriving start out-ups), WARN for possible issues that don’t crack the appliance, ERROR for precise issues, and Lethal if the program can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure vital messages and decelerate your technique. Give attention to key gatherings, condition changes, enter/output values, and demanding conclusion details within your code.
Structure your log messages Plainly and regularly. Involve context, including timestamps, ask for IDs, and function names, so it’s much easier to trace challenges in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs let you monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting This system. They’re Particularly precious in manufacturing environments wherever stepping via code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with checking dashboards.
Finally, sensible logging is about harmony and clarity. With a properly-assumed-out logging method, you may reduce the time it will take to identify problems, achieve further visibility into your applications, and improve the Total maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not just a specialized process—it is a method of investigation. To successfully recognize and correct bugs, builders will have to method the procedure similar to a detective resolving a mystery. This state of mind aids break down intricate challenges into workable parts and comply with clues logically to uncover the basis bring about.
Get started by accumulating proof. Think about the symptoms of the problem: error messages, incorrect output, or overall performance troubles. The same as a detective surveys a criminal offense scene, accumulate just as much appropriate data as you may devoid of leaping to conclusions. Use logs, examination conditions, and person stories to piece jointly a transparent image of what’s taking place.
Subsequent, form hypotheses. Ask yourself: What might be creating this behavior? Have any variations a short while ago been designed on the codebase? Has this situation occurred before less than very similar instances? The target is usually to narrow down possibilities and detect likely culprits.
Then, check your theories systematically. Attempt to recreate the condition in a very managed surroundings. If you suspect a selected operate or component, isolate it and validate if the issue persists. Similar to a detective conducting interviews, question your code concerns and Enable the final results lead you nearer to the truth.
Pay back near attention to smaller specifics. Bugs normally cover in the least predicted places—just like a lacking semicolon, an off-by-one particular error, or possibly a race condition. Be extensive and patient, resisting the urge to patch The problem without having absolutely comprehension it. Temporary fixes may possibly disguise the real challenge, only for it to resurface later on.
Last of all, maintain notes on Everything you attempted and figured out. Equally as detectives log their investigations, documenting your debugging method can help you save time for potential difficulties and assist Other folks have an understanding of your reasoning.
By pondering like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and come to be more effective at uncovering hidden difficulties in complex techniques.
Publish Checks
Creating assessments is among the most effective strategies to help your debugging abilities and All round progress performance. Tests not just assistance catch bugs early but additionally serve as a safety Internet that provides you self confidence when building variations to your codebase. A well-tested application is simpler to debug since it lets you pinpoint just wherever and when a challenge takes place.
Get started with device assessments, which center on unique functions or modules. These small, isolated checks can quickly expose irrespective of whether a selected bit of logic is Doing work as anticipated. Whenever a check fails, you instantly know where to search, considerably reducing some time expended debugging. Device exams are Particularly practical for catching regression bugs—challenges that reappear immediately after Formerly becoming mounted.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These support make certain that numerous aspects of your software function alongside one another effortlessly. They’re specially beneficial for catching bugs that occur in advanced techniques with multiple parts or providers interacting. If something breaks, your assessments can tell you which Component of the pipeline failed and under what ailments.
Creating checks also forces you to Imagine critically about your code. To check a characteristic thoroughly, you may need to understand its inputs, predicted outputs, and edge cases. This volume of knowing naturally qualified prospects to raised code construction and less bugs.
When debugging a difficulty, creating a failing exam that reproduces the bug may be a strong starting point. After the exam fails constantly, you may concentrate on repairing the bug and check out your test move when The difficulty is fixed. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, writing exams turns debugging from a discouraging guessing game into a structured and predictable method—serving to you capture more bugs, quicker and a lot more reliably.
Acquire Breaks
When debugging a tough issue, it’s uncomplicated to be immersed in the condition—staring at your screen for hours, making an attempt Resolution immediately after Alternative. But one of the most underrated debugging resources is just stepping away. Taking breaks will help you reset your head, lower irritation, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for way too prolonged, cognitive tiredness sets in. You could possibly start off overlooking noticeable glitches or misreading code you wrote just hrs previously. On this state, your brain becomes fewer economical at challenge-fixing. A short walk, a espresso crack, or simply switching to a unique process for 10–15 minutes can refresh your aim. Quite a few builders report locating the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job during the qualifications.
Breaks also support stop burnout, especially all through more time debugging sessions. Sitting down before a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent permits you to return with renewed energy and a clearer mentality. You could possibly all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you ahead of.
In the event you’re trapped, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 moment break. Use that time to maneuver close to, extend, or do some thing unrelated to code. It may well Gustavo Woltmann AI really feel counterintuitive, In particular below restricted deadlines, however it in fact leads to more rapidly and simpler debugging In the long term.
In brief, getting breaks is not a sign of weak spot—it’s a sensible method. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight That always blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Each individual bug you encounter is much more than just A brief setback—It is really an opportunity to expand for a developer. Irrespective of whether it’s a syntax error, a logic flaw, or maybe a deep architectural difficulty, each one can teach you one thing worthwhile for those who take the time to reflect and evaluate what went Mistaken.
Start out by inquiring you a few important concerns after the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved tactics like device tests, code assessments, or logging? The responses normally expose blind places as part of your workflow or knowledge and make it easier to Make more robust coding behaviors transferring ahead.
Documenting bugs can even be an outstanding practice. Hold a developer journal or keep a log where you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring difficulties or prevalent problems—which you can proactively stay away from.
In team environments, sharing Everything you've discovered from the bug with the peers can be Primarily highly effective. No matter whether it’s through a Slack information, a short create-up, or A fast expertise-sharing session, assisting others steer clear of the identical issue boosts staff effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a few of the most effective developers are usually not the ones who produce ideal code, but individuals that repeatedly discover from their problems.
In the end, Every single bug you fix adds a completely new layer for your ability established. So up coming time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Strengthening your debugging competencies can take time, practice, and persistence — although the payoff is huge. It tends to make you a far more economical, confident, and capable developer. The following time you happen to be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at what you do.